Ohkay so I read through your thread more carefully. have you tried extruding your lines on the global Z axis and then applying limited dissolve, i noticed the screenshots where there are still some bushes ending up on place where edges without intersections still have a center vertice. limited dissolve should be able to get the few that were left as well.
If you used maze generator, you can do what you want to, by simply subdividing an edge based on edge length / your preferred length…
So you’d take a line, divide its length by cell length or width ( assuming square cells here) and then add the amount of segments you’d want on a per cell basis. You could measure the cell in blender or expose it through a small script in blender. you’d convert the curves to mesh, extrude, limited dissolve, remove unwanted goemetry and then select the edge you want to measure. but just zooming in with the ruler is accurate enough most likely.
This gets the length of a selected edge: you might have to re-enter edit mode for the bmesh to get the mesh properly i noticed.
import bpy, bmesh
myobject = bpy.context.object.data
bm = bmesh.new()
bm.from_mesh(myobject)
edgelist =
for edge in bm.edges:
if edge.select == True:
edgelength = edge.calc_length()
print(edgelength)
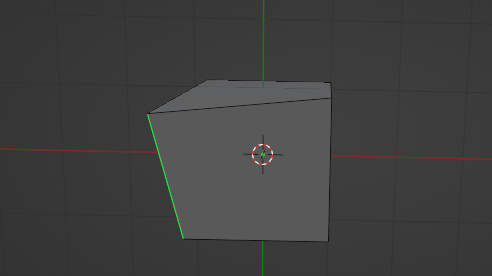
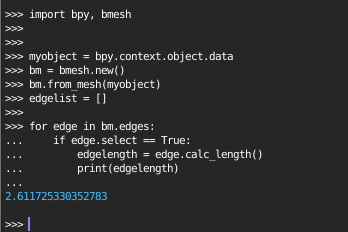
I hope this helps, you can work on that script until it does what you want, i just copy-pasted it into the console in my scripting worktab.
perhaps this is of help too:
import bpy
class SimpleOperator(bpy.types.Operator):
bl_idname = "object.simple_operator"
bl_label = "Simple Object Operator"
def execute(self, context):
import bmesh
myobject = bpy.context.object.data
bm = bmesh.new()
bm.from_mesh(myobject)
edgelist = []
for edge in bm.edges:
if edge.select == True:
edgelength = edge.calc_length()
print(edgelength)
return {'FINISHED'}
addon_keymaps =
def register():
bpy.utils.register_class(SimpleOperator)
wm = bpy.context.window_manager
kc = wm.keyconfigs.addon
if kc:
km = wm.keyconfigs.addon.keymaps.new(name='3D View', space_type='VIEW_3D')
kmi = km.keymap_items.new('object.simple_operator', type='W', value='PRESS', ctrl=True)
addon_keymaps.append((km, kmi))
def unregister():
bpy.utils.unregister_class(SimpleOperator)
# Remove the hotkey
for km, kmi in addon_keymaps:
km.keymap_items.remove(kmi)
addon_keymaps.clear()
if name == “main”:
register()
bpy.ops.object.simple_operator()
This adds that code in an operator and binds the operator to a shortcut: ‘CTRL + W’
Can see its output if you open system console:
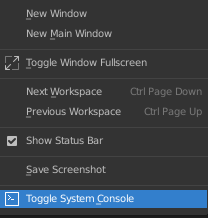