This is a bounding box.
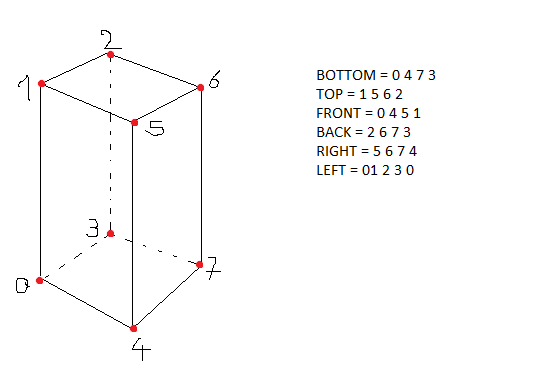
In bpy.data.objects[name].bound_box
, these points are given in this exact order. At least up to 2.79.
If the front face of your wall faces towards Y, then width is (p0 - p4).length
, and thickness is (p0 - p3).length
. Turn it the other way around if your wall faces towards X.
In upbge you could calculate these points on the fly with the min and max values of an objects cullingBox
attribute. Point 0 being (min[0], min[1])
, whereas point 4 is (max[0], min[1])
and point 3 (min[0], max[1])
. Note I’m discarding the Z as unless you also need to calculate how tall the wall is (or if it rotates weird like that), you can ignore it.
But if you’re not on upbge, or you just want to know these values beforehand rather than do the calculation at runtime, you can just get them. Write down a tool script that performs the calculation, select an object to operate on, hit the run script button, and done.
Ah, alright. Here, let me write the tool for you.
import bpy; from mathutils import Vector;
ob = bpy.context.object;
bounds = ob.bound_box;
p0, p3, p4 = Vector(bounds[0]), Vector(bounds[3]), Vector(bounds[4]);
if "thickness" not in ob.game.properties:
bpy.ops.object.game_property_new(type='FLOAT', name="thickness");
if "width" not in ob.game.properties:
bpy.ops.object.game_property_new(type='FLOAT', name="width");
ob.game.properties["thickness"].value = (p0 - p3).length;
ob.game.properties["width" ].value = (p0 - p4).length;
Now it’s a game property so you can just go object["thickness"]
when you need it.
Hope some of this is useful.
EDIT: Vector.length
is not a callable. My bad.