Would it be possible to draw anything using the gpu module under the 2d gizmos in the 3d view? I kind of assume that the answer is no (since it is drawn in POST), but I thought it would be good to ask anyway.
As an example I would like to show you how I render the rectangle that covers the gizmos but is rendered under the N-PANEL UI. I wish it was rendered under the 2d Gizmo buttons too.
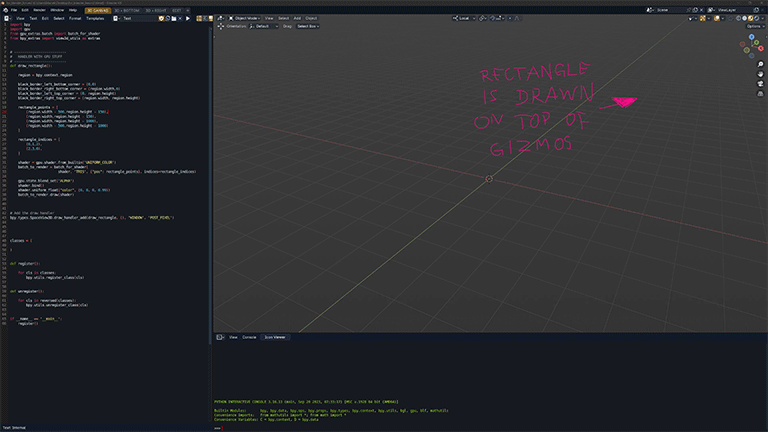
Also here is the code for the example above:
import bpy
import gpu
from gpu_extras.batch import batch_for_shader
from bpy_extras import view3d_utils as extras
# --------------------------
# HANDLER WITH GPU STUFF
# --------------------------
def draw_rectangle():
region = bpy.context.region
rectangle_points = [
(region.width - 500,region.height - 150),
(region.width,region.height - 150),
(region.width,region.height - 1000),
(region.width - 500,region.height - 1000)
]
rectangle_indices = [
(0,1,2),
(2,3,0),
]
shader = gpu.shader.from_builtin('UNIFORM_COLOR')
batch_to_render = batch_for_shader(
shader, 'TRIS', {"pos": rectangle_points}, indices=rectangle_indices)
gpu.state.blend_set('ALPHA')
shader.bind()
shader.uniform_float("color", (0, 0, 0, 0.99))
batch_to_render.draw(shader)
# Add the draw handler
bpy.types.SpaceView3D.draw_handler_add(draw_rectangle, (), 'WINDOW', 'POST_PIXEL')
classes = (
)
def register():
for cls in classes:
bpy.utils.register_class(cls)
def unregister():
for cls in reversed(classes):
bpy.utils.unregister_class(cls)
if __name__ == '__main__':
register()
Thanks a lot for any suggestions!
Maciej
AFAIK you’re pretty limited with where you can place gpu drawing in terms of depth.
From the draw_handler_add
section of the API:
draw_type (str) – Usually POST_PIXEL for 2D drawing and POST_VIEW for 3D drawing. In some cases PRE_VIEW can be used. BACKDROP can be used for backdrops in the node editor.
So you’ve got 4 options, POST_PIXEL, POST_VIEW, PRE_VIEW, BACKDROP
, and of those only PRE_VIEW
sounds like it might be helpful in your case, but I’m not sure if it will go exactly where you want.
Still, it’s something to try, and if it doesn’t work, then I think the answer is: you can’t currently do this with Python.
Thank you so much for your suggestion @SpectralVectors. I tried every single option for draw_type, but with no success. I wonder if they could add another draw_type to gpu module so you can actually render stuff under gizmos. Perhaps that could be a good suggestion for a feature.
Annotations offer the kinds of features that you’re looking for, but I’m not sure you could use them for your purposes.
They can draw to screen space, and they draws under the Navigation gizmos, and they have a sortable layer system, but they get all of that from the Grease Pencil system.
You could also try creating masks with transparent areas so that underlying gizmos don’t get covered up, but that would require external images.
@SpectralVectors - very interesting suggestions! I will explore more. I have a feeling that I might end up trying implementing UI buttons directly using GPU module to avoid 2d Gizmos altogether (not sure if it is possible to make buttons similar to 2d Gizmos using gpu module alone though). My problem is that I rely heavily on custom 2d gizmos in my addon and I am hitting the ceiling in how I can use them in combination with some custom gpu elements that I use in my Addon. Part of it is probably still lack of my experience, but I do think that there are some big limitations to 2D Gizmos too.
Thanks again for your suggestions!
If you’re looking to create your own gizmos, you can check out the bl_ui_widgets addon.
Not sure if it’s up to date, but it gives you a number of gpu drawn buttons, boxes and sliders that you can use in your addons.
(Apparently I can’t stop suggesting things
)
1 Like
Oh wow! This is great! Thank you so much for that @SpectralVectors. That is going to be super helpful to me to harness that gpu buttons. Thanks!!!